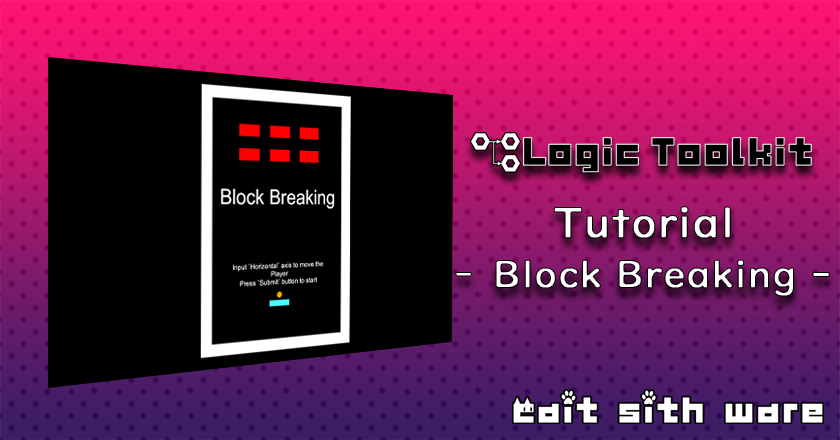
Overview
I’ll show you how to create a simple block-breaking game using Logic Toolkit.
The finished product has the same content as the sample “BlockBreaking” included with Logic Toolkit.
Prerequisite knowledge
- Basic usage of Unity editor
Content of study
- Use Logic Behavior components to control object behavior
- Use Logic Asset and Logic Player components to reuse common behaviors
- If it is a simple function, the member access script generation function allows you to configure the behavior without any coding work.
- Action nodes, State nodes, Branch nodes (flow control), etc. can coexist in the graph.
- State transition condition judgment can be shared with the Signal Evaluation node
- Share values with Blackboard variables
- Blackboard’s Data Link feature allows you to share variables between multiple objects
Shooting environment
The images and videos in this tutorial were taken in the following environment.
OS | Windows 11 |
Unity | 6000.0.0f1 |
Logic Toolkit | 1.0.0 |
Theme | Dark |
Language | English |
- Only the minimum windows in Unity are photographed.
- Explanations on adjusting the placement of the created nodes are omitted.
Preparing the project
Create a project to practice the tutorial.
Create a project
Create a project for the tutorial.
Project Name | LogicToolkit_Tutorial_BlockBreaking |
Template | 3D (Built-In Render Pipeline) |
If you have not yet downloaded the template, please download it as well.
For information on how to create projects, see “Add and remove projects in the Unity Hub” in the Unity manual.
Introduction to Logic Toolkit
Introduce Logic Toolkit to your project.
For information on installing Logic Toolkit, see “Installation steps” in the Logic Toolkit manual.
Importing the tutorial package
We have prepared a package in which a scene with block-breaking objects is created in advance.
Download “Tutorial_BlockBreaking”
LogicToolkit_Tutorial_BlockBreaking.unitypackage – Downloaded 199 times – 14.60 KBDownload the package from the link above and import it into your project.
For information on how to import packages, please refer to “Importing local asset packages” in the Unity manual.
Open scene
- Open Assets/BlockBreaking/Scenes/BlockBreaking scene
Each step
From here, we will create the behavior of individual objects.
The pages are divided, so please work from the top to the bottom.