If you haven’t prepared your project yet, please start by learning How to create a 3D Roll a Ball game.
Here we create the behavior of the Player (light blue ball).
The contents to be created are as follows:
- The Player object’s Rigidbody is moved by horizontal and vertical inputs (WASD and arrow keys).
- FixedUpdate handles the movement.
Input.GetAxis("Horizontal")
andInput.GetAxis("Vertical")
are used for input.- To move the Rigidbody, force is applied with
Rigidbody.AddForce
. - Logic Behavior is used to create the behavior.
Adding Logic Behavior to the Player
- Select the Player object
- Click the Add Component button in the Inspector window
- Select Logic Toolkit/Logic Behavior from the Component menu
- Click the Edit button in the Logic Behavior component to open the Logic Editor window
Editing Player Logic Behavior
In the Logic Editor window, you will edit the Player’s Logic Behavior.
Creating a FixedUpdate node
The best time to apply force to a Rigidbody is during FixedUpdate, so we will use the FixedUpdate node.
- Click on the graph to focus
- Press the
Space
key to open the node creation menu - Select the Scripts tab
- Select Events/Fixed Update from the list
- Confirm the node name with the
Enter
key
The node we created is called an Event node, and it is the starting point for executing other nodes.
In this case, the connected node will be executed when FixedUpdate of the Player object is called.
Creating a Rigidbody.AddForce action
Use the Logic Toolkit’s script generation feature to create a node that calls the Rigidbody.AddForce method.
- Drag and drop the Fixed Update node’s execution port to the right side of the node (where you want to create the destination node) and open the node creation menu.
- Select the Members tab.
- Enter
Rigidbody AddForce
in the search field. - Select
UnityEngine.Rigidbody
AddForce(Vector3 force) from the list. - Select Action from the node type selection menu.
- Confirm the node name with the
Enter
key.
The node we created is called an Action node, and is a node for performing simple processing.
In this case, Rigidbody.AddForce is executed.
After executing Rigidbody.AddForce, the program will immediately transition to the next node, but since nothing is connected this time, execution will end here.
The dropdown that says [Unselected] on the Members tab sets the assembly (location) where the script will be created.
If you create a node with this dropdown set to [Unselected], a default assembly with the name LogicToolkitGeneratedScripts will be created.
Normally, there’s no problem leaving the default name.
Creating a Vector3.operator* node
Calculates a 3D vector used to apply a force to a Rigidbody.
- Drag and drop the input port of the Force field of Rigidbody.AddForce to the left side of the port (where you want to create the destination node) and open the node creation menu.
- Select the Members tab.
- Enter
*
in the search field. - Select
UnityEngine.Vector3
operator *(Vector3 a, float d) from the list. - Select Compute from the node selection menu.
- Enter the node name as is and press
Enter
.
The node we created is called a Compute node, and is a node for calculating and passing values.Vector3.oeprator *(Vector3 a, float d)
returns the result of multiplying each component of the three-dimensional vector input to A by the numerical value of D.
This time, the Vector3
resulting from the calculation will be passed to the Force field of Rigidbody.AddForce.
Editing Vector3.operator*
Set the applied force to 10 this time.
- Set the value in the D field to
10
.
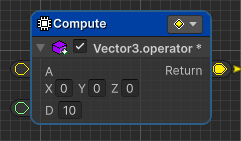
Create a new Vector3 node
Create a direction vector for applying the force.
- Drag and drop the input port of the A field of Vector3.operator * to the left side (where you want to create the destination node) and open the node creation menu.
- Select the Members tab.
- Enter
new Vector3
in the search field. - Select
UnityEngine.Vector3
new Vector3(float x, float y, float z) from the list. - Select Compute from the node selection menu.
- Confirm the node name with the
Enter
key.
Creating the Input.GetAxis node
Gets the horizontal input value.
- Drag and drop the input port of the X field of the new Vector3 node to the left side (where you want to create the destination node) and open the node creation menu.
- Select the Members tab.
- Enter
Input GetAxis
in the search field. - Select
UnityEngine.Input
GetAxis(string axisName) from the list. - Select Compute from the node type selection menu.
- Confirm the node name with the
Enter
key.
Editing the Input.GetAxis node
Set it to output the horizontal input.
- Set the string in the Axis Name field to
Horizontal
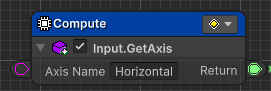
Copy and paste the Input.GetAxis node
Let’s create a node that passes the vertical input by copying and pasting an existing node.
- Select the existing Input.GetAxis Compute node
- Copy
- Windows:
Ctrl
+C
- Mac:
Cmd
+C
- Windows:
- Move the mouse cursor near the bottom of the horizontal input node
- Paste
- Windows:
Ctrl
+V
- Mac:
Cmd
+V
- Windows:
You can also copy and paste using the right-click menu of a node.
Editing the Input.GetAxis node
Set the vertical input to output.
- Set the string in the Axis Name field to
Vertical
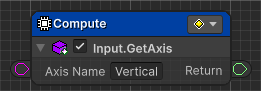
Vertical input value port connection
Connect a port to set the Vertical input value to the Z value of the new Vector3.
- Drag and drop the output port of the Return field of Input.GetAxis of Vertical to the port of the Z field of new Vector3
In fact, this method has the problem that the force applied increases when diagonal input is made.
This can be solved by inserting Vector3.ClampMagnitude
between the connection between the Output port of new Vector3 and the A port of Vector3.operator* to limit the maximum magnitude of the vector to 1
.
If this bothers you, give it a try.
Delete the Start node
We won’t be using the Start node that is provided by the beginning, so let’s delete it.
- Select the Start node
- Press the
Delete
key to delete the selected node.
Check the operation of the Player
Once you’ve done this, your graph will look like this:
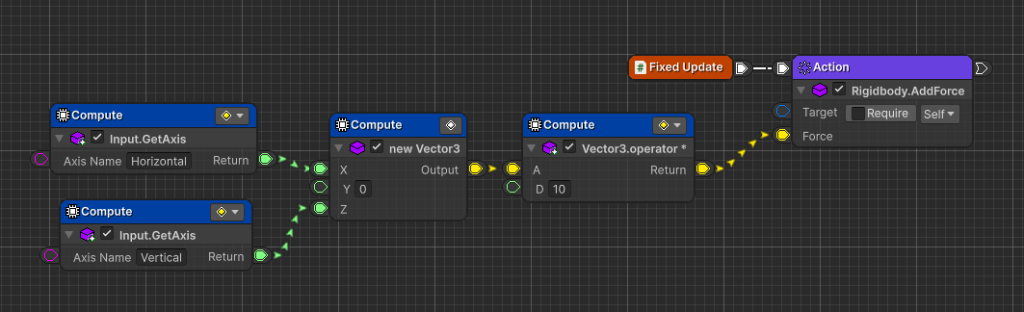
Start playing once and check the behavior of the Player (light blue ball).
- Make sure you use the
A
key orLeft arrow
key to move left - Make sure you use the
D
key orRight arrow
key to move right - Make sure you use the
W
key orUp arrow
key to move back - Make sure you use the
S
key orDown arrow
key to move forward
At this stage, you can pass through red walls and yellow cubes, but this will be implemented later, so this is not a problem.
Next time
Next time we will cover 2. Creating Main Camera behavior.
Post completion
If you would like to post on social media about the completion of the work up to this point, click here.