If you haven’t completed the previous tutorials yet, please complete them starting from Basic Usage.
This time, let’s try to get a random value using member access.
The contents are as follows.
- Create a GameObject with a Logic Behavior component.
- Open and edit the graph in the Logic Editor window.
- Use member access functions to get the value of Random.value.
Create a new scene
If you have other scenes open, it will be confusing, so create a new scene.
- Select File > New Scene from the menu.
- In the New Scene window, select Basic (URP).
- Click the Create button
Creating a GameObject with Logic Behavior
Create a GameObject with a Logic Behavior component added to the Scene.
- Click the + button in Hierarchy.
- Select Logic Toolkit > Logic Behavior from the menu.
- Confirm the name by pressing
Enter
.
Opening the Logic Editor window
Graphs of Logic Behavior components are edited in the Logic Editor window.
If the window is not displayed, select a GameObject and click the “Edit” button in the Inspector to display it.
- Select the Logic Behavior object
- Click the Edit button on the Logic Behavior component in the Inspector window.
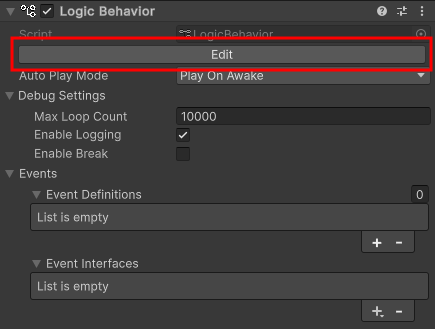
If the Logic Editor window is already displayed, the graph will switch in conjunction with selecting a GameObject in the Hierarchy window.
Create a Compute node for Random.value
Create a node that uses member access to get the Random.value.
- Right-click on an empty area of the graph view below the Start node.
- Select Create Node from the menu to open the node creation menu.
- Select the Member tab
- Type
Random.value
in the search bar - Select the value of
UnityEngine.Random
from the list. - Select Compute from the node type selection menu.
- Wait for the compilation to finish
- Confirm the node name with the
Enter key
.
About Compute Node and ActionComponent
We will explain the Compute node and ActionComponent that we created.
A Compute node is a node that performs some kind of processing and outputs the resulting value.
By outputting the result to an input port such as an Action node, you can use the processing result to perform conditional branching, etc.
The type of processing to be performed is defined in a node component called ActionComponent.
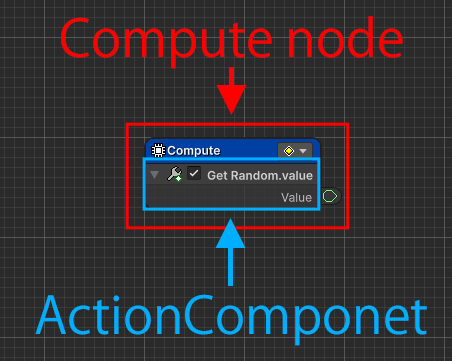
This time, we are using the member access function to create an ActionComponent that returns the result of accessing the value
property of type UnityEngine.Random
.
Do you remember that ActionComponent is also used in Action nodes?
In the Logic Toolkit, you define how to invoke a process in the node, and define the processing to be performed in the node component, allowing you to reuse node components with the same processing content in other node types.
In Compute nodes, you can use ActionComponents that output values, and processing will be performed when the value is needed in the output node.
In Action nodes, you can use any ActionComponent, regardless of whether a value is output, and processing will only be performed when a transition is made by a connection from the execution port.
Use them according to the situation.
About Member Access Feature
Most C# members (Unity API or user-defined scripts) can also be used from the graph via the member access feature.
When you select the member you want to access, a script for a node component that automatically accesses the member will be generated and made available to the node.
This time, we created an ActionComponent that accesses the value
property of the UnityEngine.Random
type and outputs the result.
Random.value is a property that returns a random float value between 0.0f
and 1.0f
.
For reference, the generated script looks like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
#pragma warning disable 0612
#pragma warning disable 0618
namespace LogicToolkit.GeneratedScripts
{
[System.Serializable]
[ScriptGenerated("Assets/Logic Toolkit/Generated Scripts/LogicToolkitGeneratedScripts/LogicToolkitGeneratedScripts.LogicToolkit_ScriptGenerator.additionalfile", 0)]
[MenuName("/LogicToolkitGeneratedScripts/UnityEngine/Random/Get Random.value")]
[MemberIconAttribute(MemberIconKind.StaticProperty)]
[DefaultRecomputeMode(RecomputeMode.Node)]
internal sealed class Generated_Get_UnityEngineːRandomːvalue : ActionComponent
{
[SerializeField]
private OutputDataPort<float> _value = new OutputDataPort<float>();
protected override void OnAction()
{
_value.SetValue(global::UnityEngine.Random.value);
}
}
}
Once created, the same script will be used whenever the same member is accessed.
The source code is automatically generated internally by the Incremental Source Generator.
(The source file is not saved under the Assets folder or elsewhere.)
The content of the generated code may differ depending on the version of the Logic Toolkit.
We won’t go into detail about the generated script here.
Just remember that this code is generated internally.
Some people may be concerned about the compilation time during automatic generation, but since there is no need to write a script and compilation does not occur on subsequent accesses, the work time is ultimately reduced.
Create a Debug.Log Action node
Let’s check the random value obtained using the Debug.Log Action node.
- Drag the Output Execute Port of the Start node
- Drop onto the graph view near the right side to open the node creation menu
- Select the Scripts tab.
- Select Actions > Debug > Debug.Log from the list.
- Confirm the node name with the
Enter key
.
Connect the ports
To output the value of Random.value to the Console, connect Debug.Log and Random.value.
- Drag the Value port of Get Random.value
- Drop it into the Message port of Debug.Log
About Data Wires
The Value port of Get Random.value and the Message port of Debug.Log are connected by a Data Wire.
(The nodes created between the ports will be explained later.)
This Data Wire is a wire (connection line) that passes values from the output port to the input port.
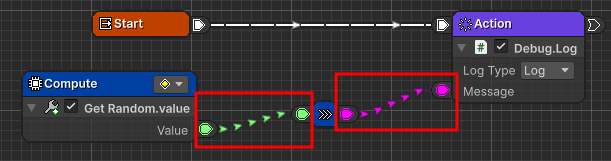
In this case, the random value obtained by Random.value is passed to the Message of Debug.Log.
About the Convert node
The node that is automatically created when you connect is called a Convert node, which automatically converts values of different types when they are connected.
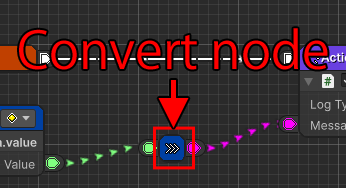
This time, the ToString node is used to convert the float type of Random.value to the string type of Message.
Play and check
Once you’ve done this, your graph will look like this:
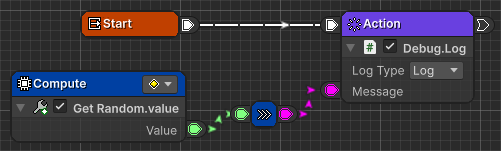
Press the play button to see how it works.
- Verify that a number between
0.0
and1.0
is displayed in the Console window. - Switch play modes several times to confirm that the numbers displayed in the Console window change.
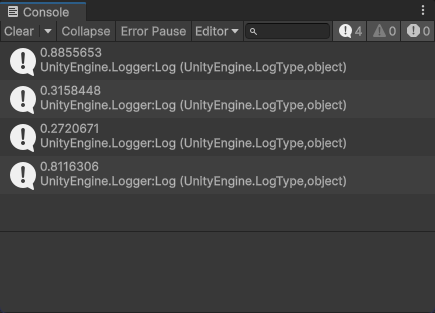
If the Console window is not visible, select Window > General > Console from the menu to display it.
If you don’t want the contents of the Console to be cleared when you start playing, as in the screenshot above, click the Clear ▼ button and turn off Clear on Play in the menu.
Save Scene
We will use what we have covered so far next time, so please save the scene.
- Press
Ctrl S
(Win) or⌘ S
(Mac) or select File > Save from the menu - Select the Assets folder
- Save it as
MemberAccess
.
Next time
Next time, we will Branch at the Branch node.
If you try the trial version and like it, you can purchase it from the Asset Store.